07-13-2018, 09:54 AM
BMOS - BitMap to OnlineSequencer v0.1 - Open Beta
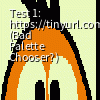
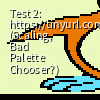
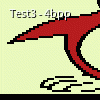
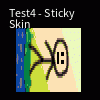
This is completely pointless, why am I doing this...
"Fork" this in the comments if you feel like a challenge, I made the code super easy to read compared to my other stuff.
UPDATED CODE (v1.3)
Code:
function bytesToInt(array, start, end) {
var result = 0;
for (i = end - 1; i >= start; i--) {
result = result * 256 + array[i];
}
return result;
}
function nearestInstrument(color) {
//var RGBColor = color.length == 4 ? color.slice(0, 3).map(x => x * color[3] / 255) : color;
var RGBColor = color.slice(0, 3);
var result;
var smallest = Infinity;
for (i = 0; i < settings.numInstruments; i++) {
var difference = 0;
for (j = 0; j < 3; j++) {
difference += (settings.instrumentColors[i][j] - RGBColor[j]) ** 2;
}
if (smallest > difference) {
smallest = difference;
result = i;
}
}
return result;
}
function drawBMP() {
SequencerView.fastGraphics = true;
//$.get(url, function (r) {
const fileData = JSON.parse('[' + localStorage.fileData + ']');
//const fileData = (new TextEncoder()).encode(r);
const imageDataOffset = bytesToInt(fileData, 10, 14);
const DIBLength = bytesToInt(fileData, 14, 18);
if (DIBLength != 40) {
return 'Not Supported: DIB: ' + DIBLength;
}
const width = bytesToInt(fileData, 18, 22);
const height = bytesToInt(fileData, 22, 26);
const heightStretch = (height - height % 72) / 72 + 1;
const bitsPerPixel = bytesToInt(fileData, 28, 30);
const compression = bytesToInt(fileData, 30, 34);
if (compression != 0) {
return 'Not Supported: Compression: ' + compression;
}
//var colors = bytesToInt(fileData, 44, 48);
colorPalette = [];
for (var i = 54; i < imageDataOffset; i += 4) {
colorPalette.push(fileData.slice(i, i + 4));
}
instrumentPalette = colorPalette.length ? [] : false;
if (instrumentPalette.length === 0) {
for (var color in colorPalette) {
instrumentPalette.push(nearestInstrument(colorPalette[color]));
}
const imageData = fileData.slice(imageDataOffset);
const jDefault = 256 / 2 ** bitsPerPixel,
jDevide = 2 ** bitsPerPixel;
var i = 0,
j = jDefault;
for (var h = height - 1; h > -1; h--) {
if (typeof piano[h / heightStretch] !== 'undefined') {
for (var w = 0; w < width; w++) {
song.addNote(new Note(song, piano[h / heightStretch], w, 1, instrumentPalette[((imageData[i] & (256 - j)) / j) % jDevide]));
j /= jDevide;
if (j < 1 || w == width - 1) {
j = jDefault;
i++;
}
}
}
}
for (var x in instrumentPalette) {
audioSystem.setInstrumentVolume(instrumentPalette[x], 0);
};
} else {
const imageData = fileData.slice(imageDataOffset);
var i = 0;
for (var h = height - 1; h > -1; h--) {
if (typeof piano[h / heightStretch] !== 'undefined') {
for (var w = 0; w < width; w++) {
song.addNote(new Note(song, piano[h / heightStretch], w, 1, nearestInstrument(imageData.slice(i, i +
bitsPerPixel / 8))));
i += bitsPerPixel / 8;
}
//i += 8 - (i % 8);
}
}
for (var x = 0; x < settings.numInstruments; x++) {
audioSystem.setInstrumentVolume(x, 0);
};
}
//})
}
This is completely pointless, why am I doing this...
"Fork" this in the comments if you feel like a challenge, I made the code super easy to read compared to my other stuff.
UPDATED CODE (v1.3)
Code:
function nearestInstrument(color) {
RGBColor = color.slice(0, 3).map(x => x * color[3] / 255);
var result;
var smallest = Infinity;
for (i = 0; i < 40; i++) {
var difference = 0;
for (j = 0; j < 3; j++) {
difference += (settings.instrumentColors[i][j] - RGBColor[j]) ** 2;
}
if (smallest > difference) {
smallest = difference;
result = i;
}
}
return result;
}
function drawImg(source) {
var img = new Image();
img.src = source;
img.onload = function() {
canvas.width = 100;
canvas.height = 50;
canvas.getContext('2d').drawImage(img, 0, 0, canvas.width, canvas.height);
var total = ':';
for (var h = canvas.height - 1; h > -1; h--) {
for (var w = 0; w < canvas.width; w++) {
var instrument = nearestInstrument(canvas.getContext('2d').getImageData(w, h, 1, 1).data);
if (instrument != 37) total += [w, piano50[h], 1, instrument].join(' ') + ';';
}
}
if (window.SequencerView) {
SequencerView.fastGraphics = true;
loadData(total);
$.each(audioSystem.instrumentVolume, x => audioSystem.setInstrumentVolume(x, 0));
} else {
console.log(total);
}
}
}
var piano50 = ["A6", "G#6", "G6", "F#6", "F6", "E6", "D#6", "D6", "C#6", "C6", "B5", "A#5", "A5", "G#5", "G5", "F#5", "F5", "E5", "D#5", "D5", "C#5", "C5", "B4", "A#4", "A4", "G#4", "G4", "F#4", "F4", "E4", "D#4", "D4", "C#4", "C4", "B3", "A#3", "A3", "G#3", "G3", "F#3", "F3", "E3", "D#3", "D3", "C#3", "C3", "B2", "A#2", "A2", "G#2"];
var settings = {instrumentColors: [[3,169,244],[255,152,0],[183,28,28],[233,30,99],[76,175,80],[33,33,33],[63,81,181],[205,220,57],[21,101,192],[130,119,23],[255,234,0],[141,110,99],[78,52,46],[255,99,99],[117,255,99],[99,224,255],[253,99,255],[255,87,34],[27,94,32],[244,67,54],[255,224,178],[117,117,117],[224,173,0],[233,249,189],[168,200,83],[13,71,161],[29,157,157],[30,197,122],[21,33,79],[5,16,47],[108,243,183],[255,112,112],[161,166,53],[234,121,0],[85,202,202],[0,128,64],[127,32,15],[0,0,0],[0,69,31],[159,15,34]]}
var canvas = document.createElement('canvas');
drawImg(document.URL);